If you have a Woocommerce site and want to increase sales on it, then I recommend trying Flash Sale. What is Flash Sale? It’s a time-limited sale accompanied by a counter that encourages your customers to make a purchase immediately, without delaying. The time limit creates a sense of urgency and can significantly increase conversion rates.
There are many free and paid plugins to implement this feature, but why install a third-party plugin when this feature is already built into your Kadence theme? Built-in theme features are usually more optimized and don’t create unnecessary load on the site, which is especially important for its speed and performance.
*Go to the Products section and create a new product or edit an existing one.
*In the product settings, specify the discounted price and set the time frame when this price will be valid.
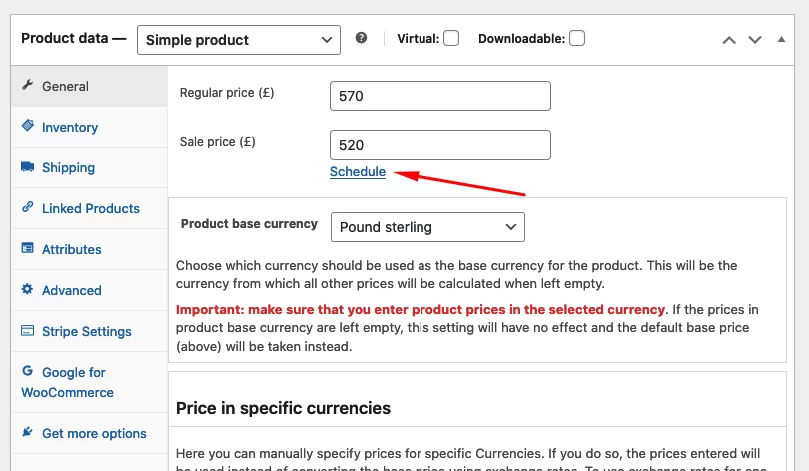
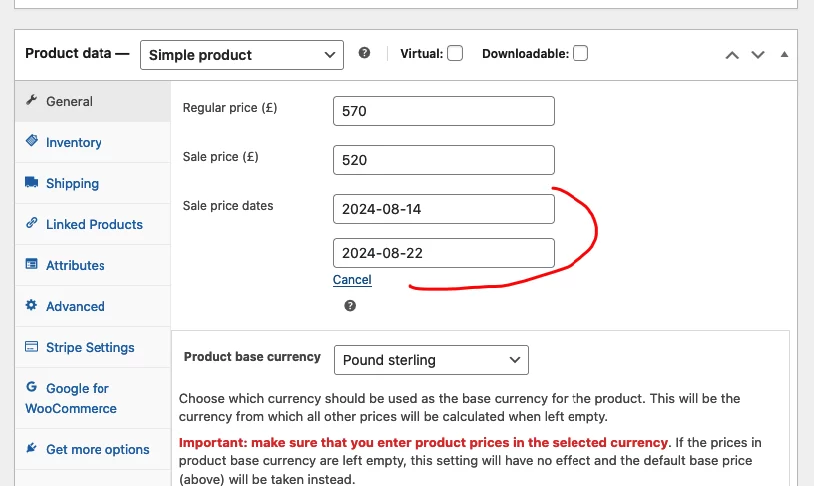
*Add the code to your child theme’s functions.php file:
//Countdown timer
add_action( 'woocommerce_single_product_summary', 'kadence_product_countdown_timer', 10 );
function kadence_product_countdown_timer() {
global $product;
if ( $product->is_on_sale() ) {
$sale_end = get_post_meta( $product->get_id(), '_sale_price_dates_to', true );
if ( $sale_end ) {
$formatted_end_date = gmdate( 'Y-m-d H:i:s', $sale_end );
echo '<div class="sale_countdown clock_jquery hasCountdown" data-time="' . esc_attr( $formatted_end_date ) . '" data-sale-id="' . esc_attr( $product->get_id() ) . '" data-format="DHMS" data-timezonetype="1" data-zone="+00:00">';
echo '<span class="sale_offer_text">Offer ends in:</span>';
echo '<span class="countdown_row countdown_show4">';
echo '<span class="countdown_section"><span class="countdown_amount">0</span><br>Day(s)</span>';
echo '<span class="countdown_section"><span class="countdown_amount">0</span><br>Hour(s)</span>';
echo '<span class="countdown_section"><span class="countdown_amount">0</span><br>Min(s)</span>';
echo '<span class="countdown_section"><span class="countdown_amount">0</span><br>Sec(s)</span>';
echo '</span></div>';
}
}
}
add_action( 'woocommerce_shop_loop_item_title', 'kadence_category_countdown_timer', 10 );
function kadence_category_countdown_timer() {
global $product;
if ( $product->is_on_sale() ) {
$sale_end = get_post_meta( $product->get_id(), '_sale_price_dates_to', true );
if ( $sale_end ) {
$formatted_end_date = gmdate( 'Y-m-d H:i:s', $sale_end );
echo '<div class="sale_countdown clock_jquery hasCountdown" data-time="' . esc_attr( $formatted_end_date ) . '" data-sale-id="' . esc_attr( $product->get_id() ) . '" data-format="DHMS" data-timezonetype="1" data-zone="+00:00">';
echo '<span class="sale_offer_text">Offer ends in:</span>';
echo '<span class="countdown_row countdown_show4">';
echo '<span class="countdown_section"><span class="countdown_amount">0</span><br>Day(s)</span>';
echo '<span class="countdown_section"><span class="countdown_amount">0</span><br>Hour(s)</span>';
echo '<span class="countdown_section"><span class="countdown_amount">0</span><br>Min(s)</span>';
echo '<span class="countdown_section"><span class="countdown_amount">0</span><br>Sec(s)</span>';
echo '</span></div>';
}
}
}
function kadence_countdown_timer_script() {
?>
<script>
document.addEventListener('DOMContentLoaded', function() {
function initializeCountdown() {
const countdownElements = document.querySelectorAll('.sale_countdown');
countdownElements.forEach(function(countdownElement) {
const endTime = new Date(countdownElement.getAttribute('data-time')).getTime();
const countdownInterval = setInterval(function() {
const currentTime = new Date().getTime();
const timeLeft = endTime - currentTime;
if (timeLeft > 0) {
const days = Math.floor(timeLeft / (1000 * 60 * 60 * 24));
const hours = Math.floor((timeLeft % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes = Math.floor((timeLeft % (1000 * 60 * 60)) / (1000 * 60));
const seconds = Math.floor((timeLeft % (1000 * 60)) / 1000);
countdownElement.querySelector('.countdown_section:nth-child(1) .countdown_amount').innerText = days;
countdownElement.querySelector('.countdown_section:nth-child(2) .countdown_amount').innerText = hours;
countdownElement.querySelector('.countdown_section:nth-child(3) .countdown_amount').innerText = minutes;
countdownElement.querySelector('.countdown_section:nth-child(4) .countdown_amount').innerText = seconds;
} else {
clearInterval(countdownInterval);
countdownElement.style.display = 'none'; // hide after end
}
}, 1000);
});
}
// Initial countdown initialization
initializeCountdown();
// Periodically check for new countdown elements
setInterval(initializeCountdown, 1000); // Check every second
});
</script>
<?php
}
add_action( 'wp_footer', 'kadence_countdown_timer_script' );
*Add the code to Additional CSS:
/*sales timer*/
@media (min-width: 768px) {
.single .summary .sale_countdown.clock_jquery.hasCountdown {
width: 375px;
}
}
.single .summary .sale_countdown.clock_jquery.hasCountdown {
background: orange;
padding: 9px;
margin-bottom: 5px;
}
.single .summary span.sale_offer_text {
font-weight: 600;
}
.product-details .sale_countdown.clock_jquery {
position: absolute;
background: orange;
top: -90px;
opacity: 0.65;
text-align: center;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
left: 0;
width: 100%;
}
.product-details .sale_countdown.clock_jquery .countdown_section {
font-size: 12px !important;
}
.product-details .sale_countdown.clock_jquery .countdown_amount {
font-size: 20px !important;
}
@media (min-width: 768px) and (max-width: 1267px) {
.product-details .sale_countdown.clock_jquery .countdown_section {
font-size: 10px !important;
padding: 0!important;
}
}
@media (max-width: 767px) {
.product-details .sale_countdown.clock_jquery .countdown_section {
font-size: 14px !important;
}
}
It’s done!
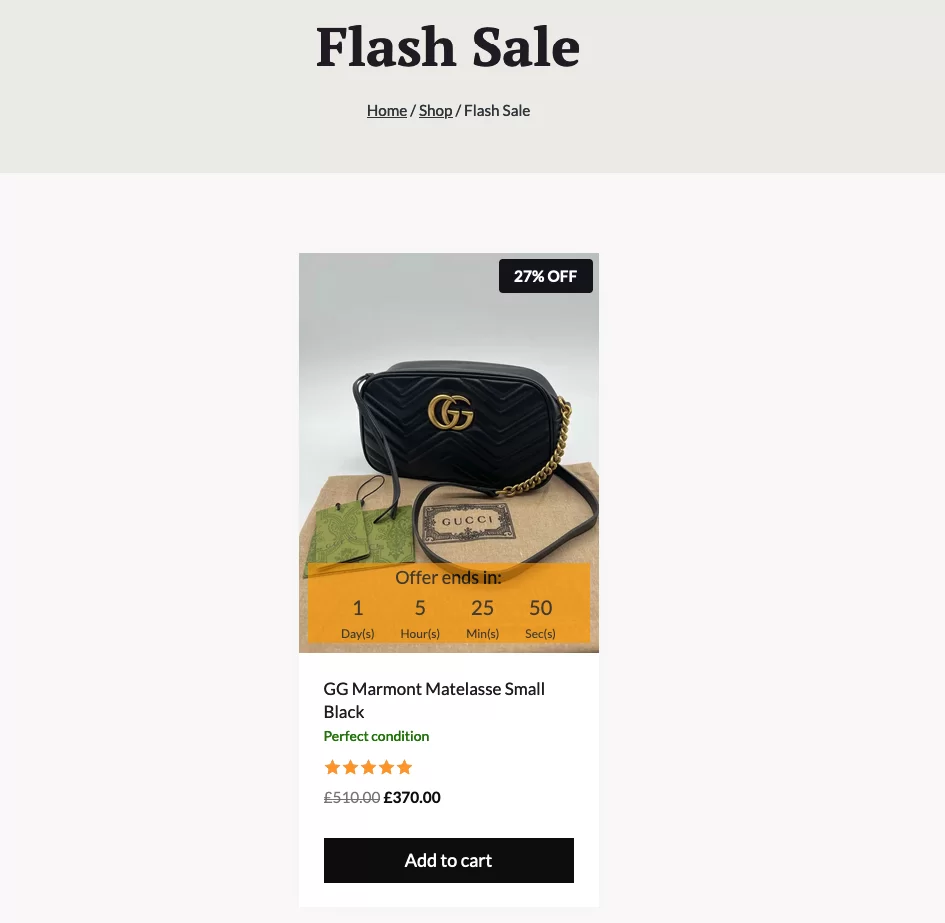
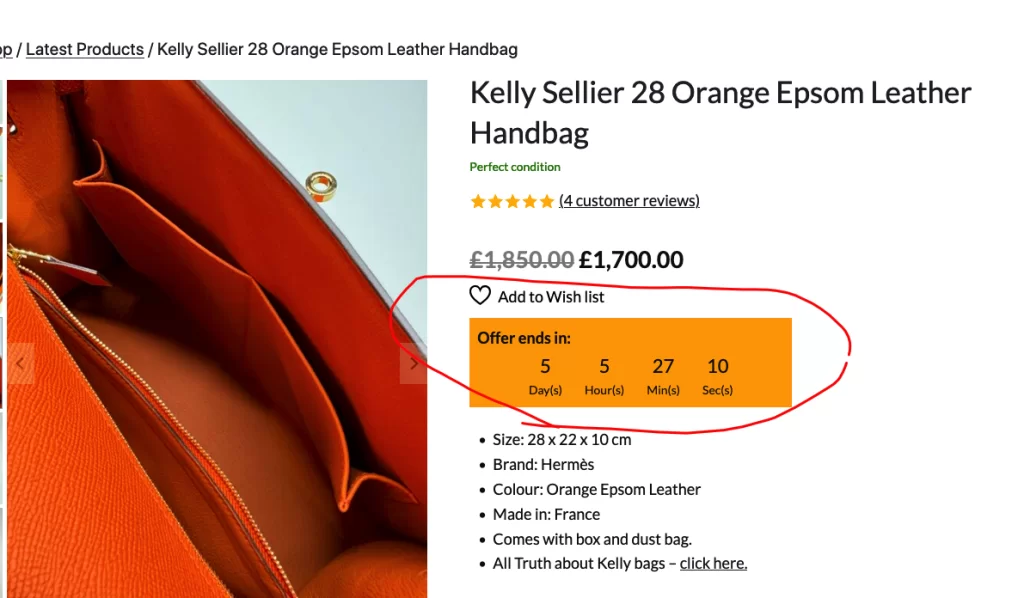
Flash Sale can be a powerful tool to increase sales on your website, especially if you properly utilize the sense of urgency and limited offers. Don’t miss the opportunity to increase the profitability of your business with minimal effort. Best of luck!
Wilbert says
Excellent post. I am experiencing a few
of these issues as well..
Louvenia says
Hi, I read your new stuff like every week. Your writing style is
awesome, keep doing what you’re doing!